With this library you will be
able to control multiple objects in a Windows NT 4.0 environment: computers,
users, groups, shares, services and printers. This library is a wrapper for the
Microsoft
ADSI (Active Directory Services Interfaces) and has been made to work under
Windows NT 4.0 Workstation or Server. It should also work under Windows 2000
running in a Windows NT 4.0 domain, or not running under a domain at all, but
this hasn't been tested.
For this wrapper
to work, you will need to install ADSI
2.5, that you can download here.
:
- 28.04.2001,
version 1.01 released.
:
- CurrentComputer as String: returns the name of the computer on which the program is running.
- CurrentDomain as String: returns the name of the primary domain, if any, that the computer
belongs to.
- CurrentUser as String: returns the user name of the current user.
- CurrentUserPrivilege as enarndUserPrivilege: returns 0 for guest, 1 for user and 2 for
administrator.
Tipically you will use this
library creating either an arndDomain object or an
arndServer object. Then you
just have to set the name of the computer or the domain you want to access to,
and you will be able to access its dependent objects. For example:
Dim SRV As arndServer, USR
As arndUser, GRP As arndGroup
Set SRV = New arndServer
SRV.Name = CurrentComputer
For Each USR In SRV.Users
Debug.Print USR.UserName
For Each GRP In USR.Groups
Debug.Print
vbTab & GRP.Name
Next
Next
Both the arndServer and the
arndDomain objects
work the same way. The arndDomain object also contains an
arndServers object,
that is a collection of the computers in the domain.
arndDomain |
arndServer |
:
- Exists as Boolean (read-only):
if you specified the name of a domain that can't be accessed or
doesn't exist, this property will be false.
- GUID as String (read-only):
returns the GUID of the domain.
- Name as String (read/write):
here you set the name of the domain you want to access.
:
- Users as arndUsers.
- Groups as arndGroups.
- Servers as arndServers.
|
:
- Division as String: returns the
company name.
- Exists as Boolean: if you
specified the name of a computer that can't be accessed or doesn't
exist, this property will be false.
- GUID as String (read-only):
returns the GUID of the computer.
- Name as String (read/write):
here you set the name of the computer you want to access.
- OperatingSystem as String
(read-only).
- OperatingSystemVersion as
String (read-only).
- Owner as String (read-only).
- Processor as String
(read-only).
- ProcessorCount as String
(read-only).
:
- Shutdown (bReboot as Boolean)
as Boolean. Use this method to shutdown or reboot a computer.
:
- Users as arndUsers.
- Groups as arndGroups.
- FileShares as arndFileShares.
- PrintQueues as arndPrintQueues.
|
The collections arndUsers,
arndGroups,
arndFileShares, arndPrintQueues and
arndServices are just that, collections. You
will be able to list, remove or add objects as you would do with any other
collection. The only things to say about them are:
- You can't add new print queues or new
services. About services, that will be fixed.
- If you add a new file share, it will be done
with the default permissions. You can't change or see permissions using this
library.
The objects that these collections contain can
also be created independently. These objects are:
arndFileShare |
arndGroup |
:
- Exists as Boolean (read-only):
only important when you use the Find method. Returns
True if the file
share exists and False if it doesn't.
- Name as String (read-only):
returns the name of the share.
- Description as String (read/write):
description of the share.
- CurrentUsers as Long
(read-only): returns how many users are connected to this share in a
certain moment.
- GUID as String (read-only).
- MaxUsers as Long (read/write):
this is the maximum number of users that can access this share at the
same time.
- Path as String (read-only):
returns the ADSI path for this share.
- Server as String (read-only):
returns the name of the computer that this shares resides on.
:
- Find (sName as String, sServer
as String) as Boolean: you can create a new arndFileShare object and
use this method to access directly a share on a computer, without
having to create an arndServer or
arndDomain object.
|
:
- Description as String
(read/write): description of the group.
- Domain as String (read-only):
it the group is global, this property will return the name of the
domain where the group is defined.
- Exists as Boolean (read-only):
only important when you use the Find method. Returns
True if the group
exists and False if it doesn't.
- GroupType as arndType
(read-only): returns [arnd Local] (1) if the group is local to the computer and
[arnd Global] (2) if
it is global in the domain.
- Name as String (read/write):
returns the name of the group. You can also change the name using this
property.
- Server as String (read-only):
if the group is local, this property will return the name of the
computer that the groups resides on.
:
- AddToLocalGroup (sGroup As
String, sServer As String) as Boolean: in a Windows NT domain, a local
group may contain not only users but also global groups, so you can
use this method and the next one for that stuff.
- RemoveFromLocalGroup (sGroup As
String, sServer As String) as Boolean: same as before.
- Find (sName As String, sParent
As String) as Boolean: you can create a new arndGroup object and use
this method to access directly a group on a computer or domain,
without having to create an arndServer or
arndDomain object.
sParent
is the name of the computer or domain that the group belongs to.
:
- User as arndUsers.
- Groups as arndGroups: only
local groups may contain global groups.
|
arndPrintQueue |
arndService |
Take into account
that this object will only work with printers that are shared over the
network, not with those that are only local to a computer.
:
- DataType as String
(read/write).
- DefaultJobPriority as Long
(read/write).
- Description as String
(read/write).
- Exists as Boolean (read-only).
- Model as String (read/write).
- Location as String
(read/write).
- Name as String (read-only).
- Path as String (read-only).
- PrintDevices as String
(read-only).
- PrintProcessor as String
(read/write).
- Priority as Long (read/write).
- Server as String (read-only).
- StartTime as Date (read/write).
- UntilTime as Date (read/write).
:
- Find (sName as String, sServer
as String) as Boolean: you can create a new arndPrintQueue object and
use this method to access directly a print queue on a computer,
without having to create an arndServer or
arndDomain object.
|
:
- ErrorControl as enarndServiceErrorControl
(read/write): returns / set what the operating system should do if the
service fails to start. Options are [arnd EC
Ignore] (1), [arnd EC Normal]
(2), [arnd EC Severe] (3) and
[arnd EC Critical] (4).
- Exists as Boolean (read-only):
only important when you use the Find method. Returns
True if the
service exists and False if it doesn't.
- DisplayName as String (read/write):
friendly display name of the service.
- LoadOrderGroup as String
(read/write): name of the load order group that this service is a
member of.
- Name as String (read-only).
- Path as String (read-only):
path and filename to the executable of this service.
- Server as String (read-only):
server that the service resides on.
- AccountName as String
(read/write): username of the account under which this service should
run. You can set the password for the account using the
SetPassword
method.
- Status as enarndServiceStatus
(read-only): [arnd SVCS Stopped] (1),
[arnd SVCS Start Pending] (2),
[arnd SVCS Stop Pending] (3),
[arnd SVCS Running] (4),
[arnd SVCS Continue Pending] (5),
[arnd SVCS Pause Pending] (6),
[arnd SVCS Paused] (7) or
[arnd SVCS Error] (8).
- ServiceType as enarndServiceType
(read/write): [arnd Kernel Driver]
(1), [arnd File System Driver] (2),
[arnd Own Process] (16), or
[arnd Share Process] (32).
- ServiceStartType as enarndServiceStartType
(read/write): [arnd SVCST Boot] (0),
[arnd SVCST System] (1),
[arnd SVCST Auto] (2),
[arnd SVCST Demand] (3) or
[arnd SVCST Disabled] (4).
:
- Continue as Boolean: if the
service is paused, this method will make the service continue working.
- Find (sName As String, Optional sServer As String)
as Boolean.
- Pause as Boolean: if the
service is running, this method will pause it.
- SetPassword (sNewPassword as
String) as Boolean: sets the password for the account under which the
service should run.
- Start as Boolean: if the
service is stopped, this method will make it start working.
- StopSvc as Boolean: if the
service is running or paused, with this method you will be able to
stop it.
:
- Dependencies: each member of
this collection is a string, that indicates the name of another
service that this service depends on.
|
arndUser |
:
- AccountDisabled as Boolean
(read/write): returns / sets if the account is disabled.
- AccountExpirationDate as Date
(read/write): date on which this account will expire, so the user will
not be able to log in.
- AccountLocked as Boolean
(read/write): returns /set if this account is locked. The operating
system locks an account when the user has tried wrong passwords a few
times.
- Description as String
(read/write): description of the account.
- Domain as String (read-only):
if the user is global, domain where it exists.
- Exists as Boolean (read-only):
only important when you use the Find method. Returns
True if the user
exists and False if it doesn't.
- FullName as String
(read/write): full name of the user.
- HomeDirDrive as String
(read/write): letter that will be mapped to the content of
HomeDirectory, so the user sees his/her home directory as a drive
letter.
- HomeDirectory as String
(read/write): user's home directory path.
- LastLogin as Date (read-only):
date and time of the last network login.
- LastLogoff as Date (read-only):
date and time of the last network logoff.
- LoginScript as String
(read/write): script that will be executed every time that the user
logs in.
- MaxStorage as Long
(read/write): maximum amount of disk storage that the user can have.
- PasswordAge as Date
(read-only): time duration of the password in use.
- PasswordCantChange as Boolean
(read/write): returns / set if the user can change the password.
- PasswordDoesntExpire as Boolean
(read/write): returns / set if the password can expire.
- PasswordExpirationDate as Date
(read/write): date and time when the password will expire.
- PasswordExpired as Date
(read-only): indicates if the password has expired.
- PasswordMinimumLength as Long
(read-only): minimum length that the password will need to have.
- PasswordRequired as Boolean
(read/write): indicated if a password needs to be set to this account.
- Profile as String (read/write):
profile that will be loaded when the user logs in.
- Server as String (read-only):
if the user is local, server where it exists.
- MustChangePasswordNextLogon as
Boolean (read/write): actually this property forces the password to
expire, so the user has to change it next time he/she logs in.
- SID as String (read-only).
- UserName as String
(read/write): you can use this property to see or change the user
name.
- UserType as arndType: returns
[arnd Local] (1) for local user and
[arnd Global] (2) for global user.
:
- AddToGroup (sGroup as String, Optional sServer
as String) as Boolean. This may be a little bit tricky: 1, if the user
is local, you don't need to specify the server name, since it can only
be added to the local computer; 2, if the user is global and you want
to add it to a global group, you don't need to specify the name of the
domain or anything, just the name of the group in the primary domain;
3, if the user is global and you want to add it to a local group in a
computer, then you need to specify the computer name.
- Find (sUserName As String, sParent As String, lUserType As arndType)
as Boolean: you can create a new arndUser object and use this method
to access directly an user on a computer or domain, without having to
create an arndServer or
arndDomain object.
sParent is the name of the
computer or domain that the user belongs to, and
lUserType must be
[arnd Local] (1) for local users and
[arnd Global] (2) for global users.
- RemoveFromGroup (sGroup as
String, Optional sServer as String) as Boolean: if the group that you
want to remove the user from is a global group (so the user is global
too), you don't need to specify anything in sServer; if the user is
local, you don't need to specify the computer either, since the user
can only be in local groups; you need to specify the server when you
want to remove a global user from a local group in a computer.
- SetPassword (sPassword as
String) as Boolean: changes the password of the user without having to
specify the old one.
:
- Groups as arndGroups: this
collection contains the groups that the user belongs to.
|
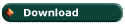 |
(63 Kb) |
© Alvaro Redondo,
2001. All Rights Reserved.
http://www.sevillaonline.com/ActiveX/